This tutorial demonstrates the process of obtaining the client IP address using PHP. It covers scenarios where capturing and storing the client IP address in a database via PHP is necessary. Additionally, the tutorial explores actions contingent upon the user’s IP address, including implementing security measures like blocking users after multiple invalid login attempts based on their IP address. Following the discussion on PHP IP address handling, the next focus is on the technicalities of PayPal integration in PHP, enhancing PHP’s utility in online transaction processing.
Techniques for Acquiring Client IP Address Using PHP
Multiple methods exist for obtaining the client IP address in PHP, and this discussion will cover more than one of these techniques.
Method 1:
PHP offers a built-in superglobal variable, $_SERVER, containing valuable information. This variable can be utilized to retrieve the client IP address in PHP. The following PHP code returns the user’s IP address:
<?php
echo $_SERVER['REMOTE_ADDR'];
?>
However, this approach might yield an incorrect IP address if the user employs a proxy. An alternative solution is available to address this issue.
Method 2:
A custom PHP function can be employed to ascertain the actual IP address of a user in PHP, even when a proxy is used. This function also checks for proxy usage, which might involve multiple IP addresses, and selects the first IP address as the most reliable one.
<?php
// Get the Client IP Address PHP Function
function get_ip_address() {
$ip_address = '';
if (!empty($_SERVER['HTTP_CLIENT_IP'])){
$ip_address = $_SERVER['HTTP_CLIENT_IP']; // Get the shared IP Address
}else if(!empty($_SERVER['HTTP_X_FORWARDED_FOR'])){
//Check if the proxy is used for IP/IPs
// Split if multiple IP addresses exist and get the last IP address
if (strpos($_SERVER['HTTP_X_FORWARDED_FOR'], ',') !== false) {
$multiple_ips = explode(",", $_SERVER['HTTP_X_FORWARDED_FOR']);
$ip_address = trim(current($multiple_ips));
}else{
$ip_address = $_SERVER['HTTP_X_FORWARDED_FOR'];
}
}else if(!empty($_SERVER['HTTP_X_FORWARDED'])){
$ip_address = $_SERVER['HTTP_X_FORWARDED'];
}else if(!empty($_SERVER['HTTP_FORWARDED_FOR'])){
$ip_address = $_SERVER['HTTP_FORWARDED_FOR'];
}else if(!empty($_SERVER['HTTP_FORWARDED'])){
$ip_address = $_SERVER['HTTP_FORWARDED'];
}else{
$ip_address = $_SERVER['REMOTE_ADDR'];
}
return $ip_address;
}
// Print client IP address
$ip_address = get_ip_address();
echo "Your IP Address is: ". $ip_address;
?>
This process successfully retrieves the IP address of a user or client. By utilizing this function, one can easily obtain the client IP address using PHP. For demonstration, the client IP address is displayed.
IP Address Validation Process
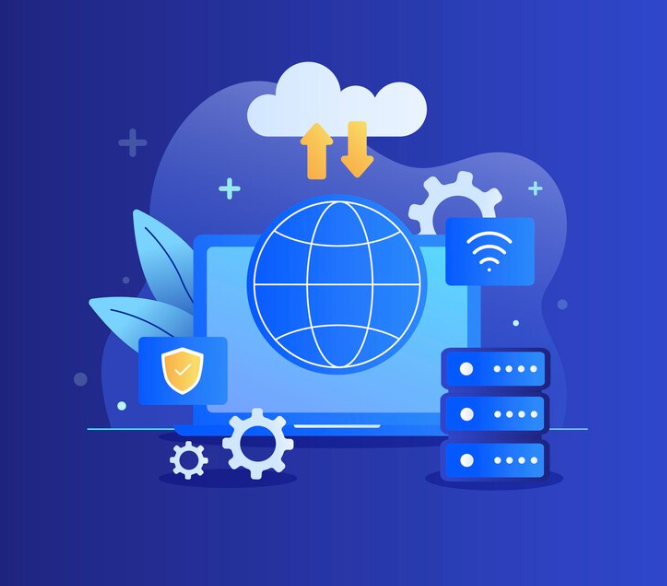
Ensuring the validity of an IP address is a critical step, particularly when using PHP functions in environments like localhost, where the output might be “::1” instead of the actual IP address.
- In some instances, there is a possibility of receiving invalid IP addresses from users;
- Consequently, it’s advisable to verify the IP address’s validity before utilizing or storing it.
The provided PHP function is designed to assess the validity of both IPv4 and IPv6 addresses. Additionally, it includes checks to confirm that the IP address does not belong to private or reserved network ranges.
<?php
// Get the Client IP Address PHP Function
function get_ip_address() {
$ip_address = '';
if (!empty($_SERVER['HTTP_CLIENT_IP'])){
$ip_address = $_SERVER['HTTP_CLIENT_IP']; // Get the shared IP Address
}else if(!empty($_SERVER['HTTP_X_FORWARDED_FOR'])){
//Check if the proxy is used for IP/IPs
// Split if multiple IP addresses exist and get the last IP address
if (strpos($_SERVER['HTTP_X_FORWARDED_FOR'], ',') !== false) {
$multiple_ips = explode(",", $_SERVER['HTTP_X_FORWARDED_FOR']);
$ip_address = trim(current($multiple_ips));
}else{
$ip_address = $_SERVER['HTTP_X_FORWARDED_FOR'];
}
}else if(!empty($_SERVER['HTTP_X_FORWARDED'])){
$ip_address = $_SERVER['HTTP_X_FORWARDED'];
}else if(!empty($_SERVER['HTTP_FORWARDED_FOR'])){
$ip_address = $_SERVER['HTTP_FORWARDED_FOR'];
}else if(!empty($_SERVER['HTTP_FORWARDED'])){
$ip_address = $_SERVER['HTTP_FORWARDED'];
}else{
$ip_address = $_SERVER['REMOTE_ADDR'];
}
return $ip_address;
}
// Print client IP address
$ip_address = get_ip_address();
echo "Your IP Address is: ". $ip_address;
?>
Privacy Considerations in IP Data Collection
The IP address of a client constitutes private information. It is imperative to clearly state in the privacy policy the practice of collecting client IP addresses, along with the reasons for this data collection.
Conclusion
Acquiring client IP addresses using PHP may be an invaluable tool for enhancing website security, providing personalized user experiences, and gathering insightful analytics. However, it is critical to handle such data responsibly due to privacy concerns. This detailed guide helps you understand the process better, empowering you to utilize PHP for tracking client IP addresses effectively.